Summary: in this tutorial, you’ll learn how to use the Flutter Wrap
widget that displays its child widgets in multiple horizontal or vertical runs.
Introduction to the Flutter wrap
The Row
and Column
widgets lay out child widgets and distribute the space between them horizontally or vertically. However, these widgets do not scroll. In other words, if there is not enough space for their children, an overflow error will occur.
For example, the following uses the Row
widget to display multiple Chip
widgets in a horizontal array. However, the Row
widget does not have enough space for all the chips, and an overflow error occurs:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
body: SafeArea(
child: Row(
children: [
Chip(
avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('EL')),
label: const Text('Elon Musk'),
),
Chip(
avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('BG')),
label: const Text('Bill Gates'),
),
Chip(
avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('MZ')),
label: const Text('Mark Zuckerberg'),
),
Chip(
avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('JB')),
label: const Text('Jeff Bezos'),
),
],
)
),
),
);
}
}
Code language: Dart (dart)
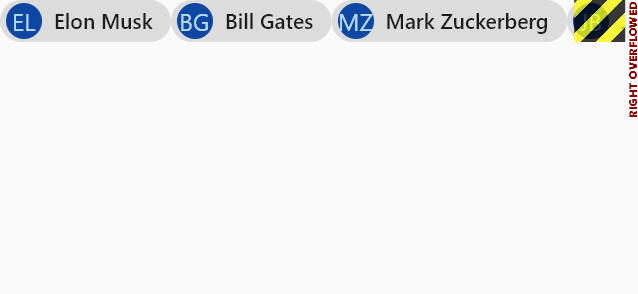
To solve this problem, you can use the Wrap
widget.
The Wrap
widget places its child widget adjacent to the previous child in the main axis and leaves space between them.
If there is not sufficient space to fit all the children, the Wrap
widget creates a new run adjacent to the existing children in the cross axis.
Like Column
and Row
widgets, the Wrap
widget also has two axes: main and cross.
By default, the main axis is from left to right and the cross axis is from top to bottom. The direction
property specifies which direction to use as the main axis. It defaults to Axis.horizontal
.
If you set the direction to Axis.vertical
, the main axis is from top to bottom and the cross axis is from left to right.
Besides the direction
property, the Wrap
widget has the following useful properties:
space
– specifies the space between children in the main axis.runSpacing
– specifies the space between runs in the cross axis.
For example, the following replaces the Row
widget with the Wrap
widget:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
body: SafeArea(
child: Wrap(
spacing: 8.0, // gap between adjacent chips
runSpacing: 4.0, // gap between lines
children: <Widget>[
Chip(
avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('EL')),
label: const Text('Elon Musk'),
),
Chip(
avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('BG')),
label: const Text('Bill Gates'),
),
Chip(
avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('MZ')),
label: const Text('Mark Zuckerberg'),
),
Chip(
avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('JB')),
label: const Text('Jeff Bezos'),
),
],
)
),
),
);
}
}
Code language: Dart (dart)
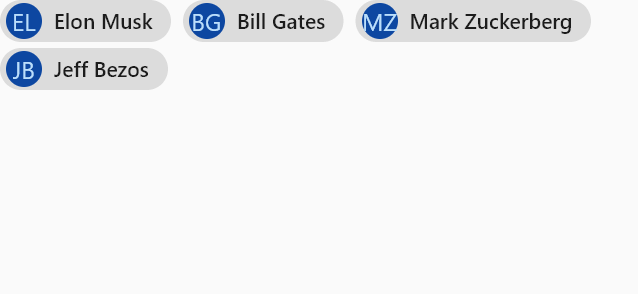
If you change the direction property to Axis.vertical
, the Chip
widgets will run in the vertical direction:
direction: Axis.horizontal
Code language: Dart (dart)
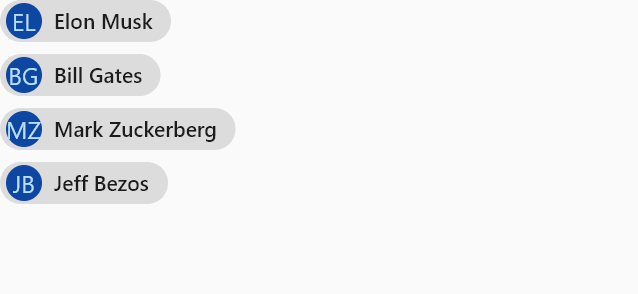
Summary
- Use the Flutter Wrap widget to display its children in multiple horizontal or vertical runs.